HTML Interactive Form Validation
Creating forms in HTML has always been complicated. You first need to write the correct markup, then you need to make sure each field has a valid value before submitting, and finally you need to inform the user when there is a problem.
Thankfully, new features were introduced in HTML5 to make this a lot easier. In particular, the form controls were extended to support constraints, allowing the browser to validate form content client-side, without the need for JavaScript.
WebKit already had partial support for this. It was possible to use attributes on form controls to describe constraints and then query the validity of a form control or a whole form using the checkValidity()
API in JavaScript. It was also possible to understand which constraint was violated using the ValidityState API.
However, WebKit did not support HTML interactive form validation, which occurs on form submission (unless the novalidate
attribute is set on the <form>
element) or using the reportValidity()
API. We are pleased to announce that this is now implemented in WebKit and enabled in Safari Technology Preview 19. Upon interactive form validation, WebKit will now check the validity of all form controls in the form. If there is at least one form control that violates a constraint, WebKit will focus the first one, scroll it into view, and display a bubble near it with a message explaining what the problem is.
Validation Constraints
Input type
Some input types have intrinsic constraints. Setting the type to “email”, “number” or “URL” will automatically check that the value is a valid email, number or URL, e.g.:
<input type="email">
Validation attributes
The following attributes can be used to describe constraints on form controls:
- required: Indicates that the user must enter a value.
- pattern=“[a-z]”: Indicates that the user must enter a value that matches the JavaScript regular expression provided.
- minlength=x: Indicates that the user must enter a value that has at least x characters.
- maxlength=y: Indicates that the user must enter at most y characters.
- min=x: Indicates that the user must enter a value that is greater than or equal to x.
- max=y: Indicates that the user must enter a value that is less than or equal to y.
- step=x: Indicates that the user must enter a value that is min + an integral multiple of x.
Constraint validation
Constraint validation can happen in several ways:
- It is possible to call checkValidity() on a form element or on a specific form control. This will simply return false if any constraint is violated, and true otherwise. It will also fire an event named “invalid” at the invalid element(s). It is possible to check which constraint was violated using the ValidityState object which is exposed via the “validity” attribute on form controls.
- It is possible to call reportValidity() on a form element or on a specific form control. This triggers interactive validation of the constraints. In addition to doing the same things as
checkValidity()
,reportValidity()
will also focus the first invalid element, scroll it into view and show a bubble near it with a message explaining the problem. - Interactive form validation also happens when submitting the form, unless the “novalidate” attribute is set on the
<form>
element.
Custom constraints
It is possible to implement more complex validation constraints or provide more useful error messages for an invalid input using JavaScript to do the validation and then using the setCustomValidity()
API.
JavaScript can be triggered by listening for a given event (e.g. onchange, oninput, …) on a form control. The executed JavaScript code can then validate the form control’s data and update the control’s error message using setCustomValidity()
:
<label for="feeling">Feeling:</label>
<input id="feeling" type="text" oninput="validateFeeling(this)">
<script>
function validateFeeling(input) {
if (input.value == "good" || input.value == "fine" || input.value == "tired") {
input.setCustomValidity('"' + input.value + '" is not a feeling');
} else {
// The data is valid, reset the error message.
input.setCustomValidity('');
}
}
</script>
Validation message bubble
Upon interactive form validation, a bubble with a message explaining the problem will show near the first form control that has invalid data, like so:
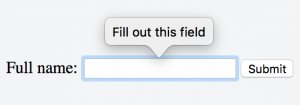
There is a default set of localized validation messages for builtin constraints. If you wish to customize the validation message, consider using the setCustomValidity()
API. Note that WebKit also supports the JavaScript internationalization API which can help with the localization of custom validation messages.
Conclusion
HTML interactive form validation is now supported in WebKit and enabled by default in Safari Technology Preview 19. Please give our live demo a try and experiment with the feature. Bug reports are welcome.